Learn ASP.NET MVC 5
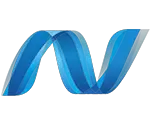
ASP.NET is a free web framework for building websites and web applications on .NET Framework using HTML, CSS, and JavaScript. ASP.NET MVC 5 is a web framework based on Model-View-Controller (MVC) architecture. Developers can build dynamic web applications using ASP.NET MVC framework that enables a clean separation of concerns, fast development, and TDD friendly.
Basics
- Overview of MVC Architecture
- ASP.NET MVC Framework Version History
- Create First MVC App
- MVC Folder Structure
- Routing in ASP.NET MVC
- Filters in ASP.NET MVC
- ActionFilter Attributes
- What is Bundling in ASP.NET MVC?
- ScriptBundle
- StyleBundle
- Area in ASP.NET MVC
- ASP.NET MVC Learning Resources
Create HTML Controls in MVC
Model, View, & Controller
- Controllers in ASP.NET MVC
- Action methods in Controller
- Action Selector Attributes
- HttpGet, HttpPost, HttpPut
- Model in MVC
- View in MVC
- Razor View Syntax
- Integrate Model, View & Controller
- Binding Model to View and Controller
- Create View To Edit Data
- What is Layout View?
- Create Layout View in ASP.NET MVC
- What is Partial View?
- What is ViewBag?
- What is ViewData?
- What is TempData?
- Implement Validations in ASP.NET MVC Form
- Use ValidationSummary to Display Error Summary
- Exception Handling in ASP.NET MVC
ASP.NET MVC Test |
MVC Test |
ASP.NET MVC Articles
- How to upload files in ASP.NET MVC?
- Redirect from HTTP to HTTPS in ASP.NET
- Redirect non-www to www domain in ASP.NET
- Build e-commerce application on .NET Framework
- How to create a custom filter in ASP.NET MVC?
- How to use web.config customErrors in ASP.NET MVC?
- How to display a custom error page with error code using httpErrors in ASP.NET MVC?
- Difference between Html.Partial() and Html.RenderPartial() in ASP.NET MVC
- Difference between Html.RenderBody() and Html.RenderSection() in ASP.NET MVC
- What is RouteData in ASP.NET MVC?
- How to set image path in StyleBundle?
- How to pre-compile razor view in ASP.NET MVC?
- How to enable bundling and minification in ASP.NET MVC?
- How to enable client side validation in ASP.NET MVC?
- How to display an error message using ValidationSummary in ASP.NET MVC?
- How to define a custom action selector in ASP.NET MVC?
- How to bind a model to a partial view in ASP.NET MVC?
- View in ASP.NET MVC