First C# Program
Here, you will learn to create a simple console application in C# and understand the basic building blocks of a console application.
C# can be used in a window-based, web-based, or console application. To start with, we will create a console application to work with C#.
Open Visual Studio (2017 or later) installed on your local machine. Click on File -> New Project... from the top menu, as shown below.
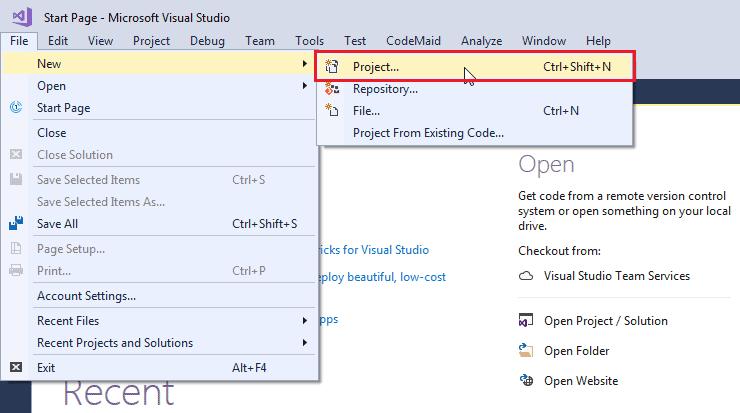
From the New Project popup, shown below, select Visual C# in the left side panel and select the Console App in the right-side panel.
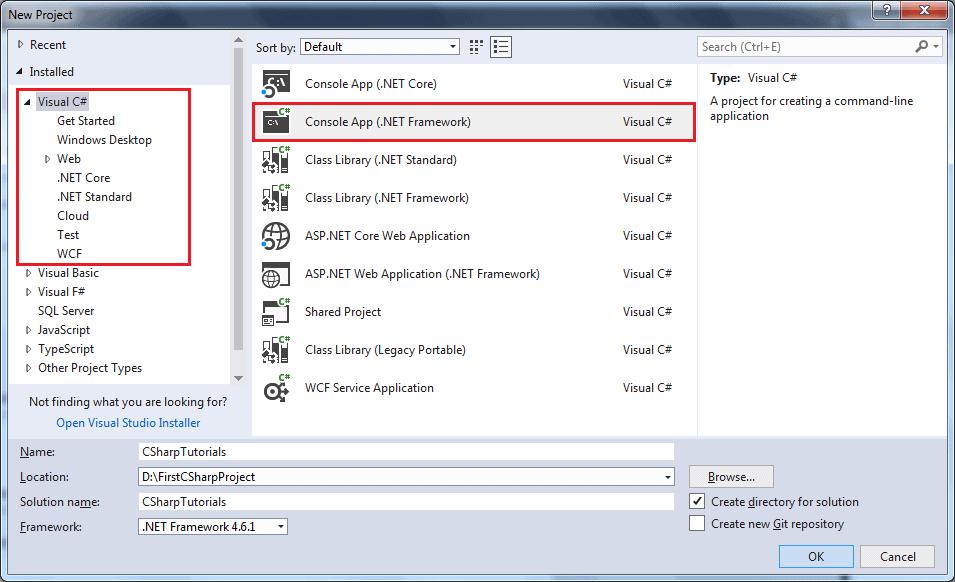
In the name section, give any appropriate project name, a location where you want to create all the project files, and the name of the project solution.
Click OK to create the console project. Program.cs will be created as default a C# file in Visual Studio where you can write your C# code in Program class, as shown below. (The .cs is a file extension for C# file.)
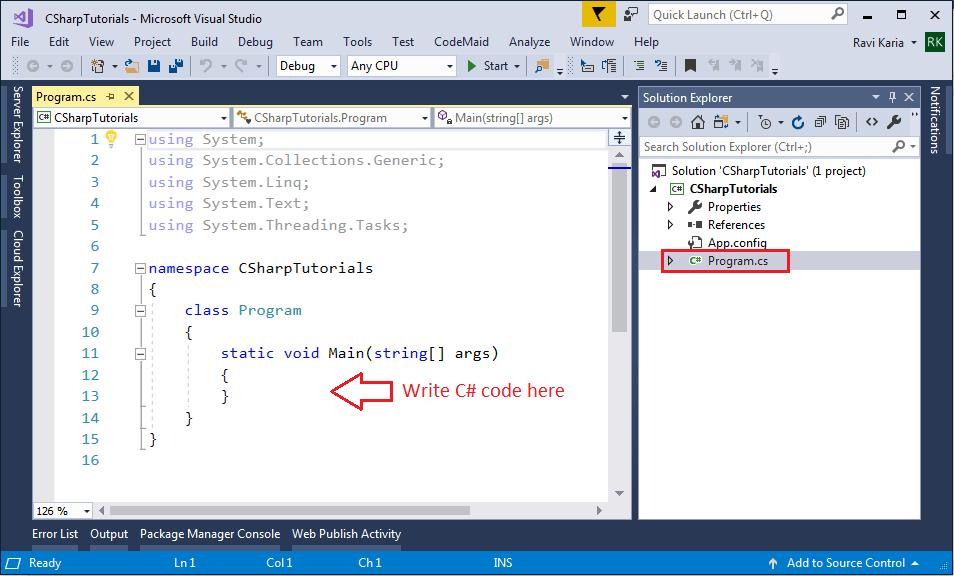
Every console application starts from the Main()
method of the Program
class. The following example displays "Hello World!!" on the console.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CSharpTutorials
{
class Program
{
static void Main(string[] args)
{
string message = "Hello World!!";
Console.WriteLine(message);
}
}
}
The following image illustrates the important parts of the above example.
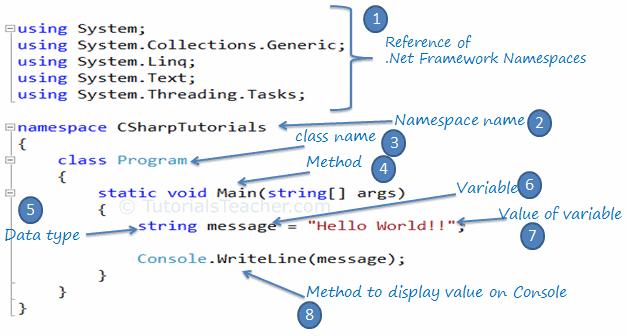
Let's understand the above C# structure.
- Every .NET application takes the reference of the necessary .NET framework namespaces that it is planning to use with the
using
keyword, e.g.,using System.Text
. - Declare the namespace for the current class using the
namespace
keyword, e.g.,namespace CSharpTutorials.FirstProgram
- We then declared a class using the
class
keyword:class Program
- The
Main()
is a method ofProgram
class is the entry point of the console application. String
is a data type.- A
message
is a variable that holds the value of a specified data type. "Hello World!!"
is the value of the message variable.- The
Console.WriteLine()
is a static method, which is used to display a text on the console.
Compile and Run C# Program
To see the output of the above C# program, we have to compile it and run it by pressing Ctrl + F5 or clicking the Run button or by clicking the "Debug" menu and clicking "Start Without Debugging". You will see the following output in the console:
So this is the basic code items that you will probably use in every C# code.