JavaScript Hoisting
Hoisting is a concept in JavaScript, not a feature. In other scripting or server side languages, variables or functions must be declared before using it.
In JavaScript, variable and function names can be used before declaring it. The JavaScript compiler moves all the declarations of variables and functions at the top so that there will not be any error. This is called hoisting.
x = 1;
alert('x = ' + x); // display x = 1
var x;
The following figure illustrates hoisting.
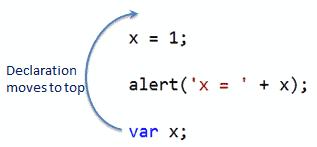
Also, a variable can be assigned to another variable as shown below.
x = 1;
y = x;
alert('x = ' + x);
alert('y = ' + y);
var x;
var y;
Hoisting is only possible with declaration but not the initialization. JavaScript will not move variables that are declared and initialized in a single line.
alert('x = ' + x); // display x = undefined
var x = 1;
As you can see in the above example, value of x will be undefined because var x = 1 is not hoisted.
Hoisting of Function
JavaScript compiler moves the function definition at the top in the same way as variable declaration.
alert(Sum(5, 5)); // 10
function Sum(val1, val2)
{
return val1 + val2;
}
Please note that JavaScript compiler does not move function expression.
Add(5, 5); // error
var Add = function Sum(val1, val2)
{
return val1 + val2;
}
Hoisting Functions Before Variables
JavaScript compiler moves a function's definition before variable declaration. The following example proves it.
alert(UseMe);
var UseMe;
function UseMe()
{
alert("UseMe function called");
}
As per above example, it will display UseMe function definition. So the function moves before variables.
- JavaScript compiler moves variables and function declaration to the top and this is called hoisting.
- Only variable declarations move to the top, not the initialization.
- Functions definition moves first before variables.