JavaScript Arrays: Create, Access, Add & Remove Elements
We have learned that a variable can hold only one value. We cannot assign multiple values to a single variable. JavaScript array is a special type of variable, which can store multiple values using a special syntax.
The following declares an array with five numeric values.
let numArr = [10, 20, 30, 40, 50];
In the above array, numArr
is the name of an array variable. Multiple values are assigned to it by separating them using a comma inside square brackets as [10, 20, 30, 40, 50]
.
Thus, the numArr
variable stores five numeric values.
The numArr
array is created using the literal syntax and it is the preferred way of creating arrays.
Another way of creating arrays is using the Array()
constructor, as shown below.
let numArr = new Array(10, 20, 30, 40, 50);
Every value is associated with a numeric index starting with 0. The following figure illustrates how an array stores values.
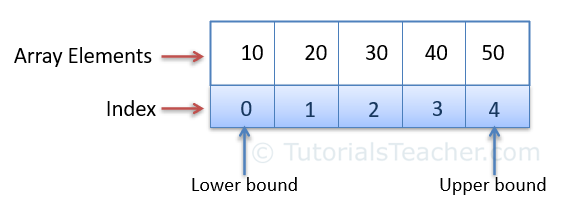
The following are some more examples of arrays that store different types of data.
let stringArray = ["one", "two", "three"];
let numericArray = [1, 2, 3, 4];
let decimalArray = [1.1, 1.2, 1.3];
let booleanArray = [true, false, false, true];
It is not required to store the same type of values in an array. It can store values of different types as well.
let data = [1, "Steve", "DC", true, 255000, 5.5];
Get Size of an Array
Use the length
property to get the total number of elements in an array. It changes as and when you add or remove elements from the array.
let cities = ["Mumbai", "New York", "Paris", "Sydney"];
console.log(cities.length); //4
cities[4] = "Delhi";
console.log(cities.length); //5
Accessing Array Elements
Array elements (values) can be accessed using an index. Specify an index in square brackets with the array name to access the element at a particular index like arrayName[index]
. Note that the index of an array starts from zero.
let numArr = [10, 20, 30, 40, 50];
console.log(numArr[0]); // 10
console.log(numArr[1]); // 20
console.log(numArr[2]); // 30
console.log(numArr[3]); // 40
console.log(numArr[4]); // 50
let cities = ["Mumbai", "New York", "Paris", "Sydney"];
console.log(cities[0]); // "Mumbai"
console.log(cities[1]); // "New York"
console.log(cities[2]); // "Paris"
console.log(cities[3]); // "Sydney"
//accessing element from nonexistance index
console.log(cities[4]); // undefined
For the new browsers, you can use the arr.at(pos)
method to get the element from the specified index.
This is the same as arr[index]
except that the at()
returns an element from the last element if the specified index is negative.
let numArr = [10, 20, 30, 40, 50];
console.log(numArr.at(0)); // 10
console.log(numArr.at(1)); // 20
console.log(numArr.at(2)); // 30
console.log(numArr.at(3)); // 40
console.log(numArr.at(4)); // 50
console.log(numArr.at(5)); // undefined
//passing negative index
console.log(numArr.at(-1)); // 50
console.log(numArr.at(-2)); // 40
console.log(numArr.at(-3)); // 30
console.log(numArr.at(-4)); // 20
console.log(numArr.at(-5)); // 10
console.log(numArr.at(-6)); // undefined
You can iterate an array using Array.forEach()
, for, for-of, and for-in loop, as shown below.
let numArr = [10, 20, 30, 40, 50];
numArr.forEach(i => console.log(i)); //prints all elements
for(let i=0; i<numArr.length; i++)
console.log(numArr[i]);
for(let i of numArr)
console.log(i);
for(let i in numArr)
console.log(numArr[i]);
Update Array Elements
You can update the elements of an array at a particular index using arrayName[index] = new_value
syntax.
let cities = ["Mumbai", "New York", "Paris", "Sydney"];
cities[0] = "Delhi";
cities[1] = "Los angeles";
console.log(cities); //["Delhi", "Los angeles", "Paris", "Sydney"]
Adding New Elements
You can add new elements using arrayName[index] = new_value
syntax.
Just make sure that the index is greater than the last index. If you specify an existing index then it will update the value.
let cities = ["Mumbai", "New York", "Paris", "Sydney"];
cities[4] = "Delhi"; //add new element at last
console.log(cities); //["Mumbai", "New York", "Paris", "Sydney", "Delhi"]
cities[cities.length] = "London";//use length property to specify last index
console.log(cities); //["Mumbai", "New York", "Paris", "Sydney", "Delhi", "London"]
cities[9] = "Pune";
console.log(cities); //["Mumbai", "New York", "Paris", "Sydney", "Delhi", "Londen", undefined, undefined, undefined, "Pune"]
In the above example, cities[9] = "Pune"
adds "Pune"
at 9th index and all other non-declared indexes as undefined.
The recommended way of adding elements at the end is using the push()
method. It adds an element at the end of an array.
let cities = ["Mumbai", "New York", "Paris", "Sydney"];
cities.push("Delhi"); //add new element at last
console.log(cities); //["Mumbai", "New York", "Paris", "Sydney", "Delhi"]
Use the unshift()
method to add an element to the beginning of an array.
let cities = ["Mumbai", "New York", "Paris", "Sydney"];
cities.unshift("Delhi"); //adds new element at the beginning
console.log(cities); //["Delhi", "Mumbai", "New York", "Paris", "Sydney"]
cities.unshift("London", "Pune"); //adds new element at the beginning
console.log(cities); //["London", "Pune", "Delhi", "Mumbai", "New York", "Paris", "Sydney"]
Remove Array Elements
The pop()
method returns the last element and removes it from the array.
let cities = ["Mumbai", "New York", "Paris", "Sydney"];
let removedCity = cities.pop(); //returns and removes the last element
console.log(cities); //["Mumbai", "New York", "Paris"]
The shift()
method returns the first element and removes it from the array.
let cities = ["Mumbai", "New York", "Paris", "Sydney"];
let removedCity = cities.shift(); //returns first element and removes it from array
console.log(cities); //["New York", "Paris", "Sydney"]
You cannot remove middle elements from an array. You will have to create a new array from an existing array without the element you do not want, as shown below.
let cities = ["Mumbai", "New York", "Paris", "Sydney"];
let cityToBeRemoved = "Paris";
let mycities = cities.filter(function(item) {
return item !== cityToBeRemoved
})
console.log(mycities); //["Mumbai", "New York", "Sydney"]
console.log(cities); //["Mumbai", "New York", "Paris", "Sydney"]
Learn about array methods and properties in the next chapter.